Log agents
Describes how to track agents using Opik
When working with agents, it can become challenging to track the flow of the agent and its interactions with the environment. Opik provides a way to track both the agent definition and it’s flow.
Opik includes an integration with many popular Agent frameworks (LangGrah, LLamaIndex)
and can also be used to log agents manually using the @track
decorator.
We are working on improving Opik’s support for agent workflows, if you have any ideas or suggestions for the roadmap, you can create a new Feature Request issue in the Opik Github repo or book a call with the Opik team: Talk to the Opik team.
Track agent execution
You can track the agent execution by using either one of Opik’s integrations or the @track
decorator:
LangGraph
Haystack
LLamaIndex
Manual Tracking
Once the agent is executed, you will be able to view the execution flow in the Opik dashboard. In the trace sidebar, you will be able to view each step that has been executed in chronological order:
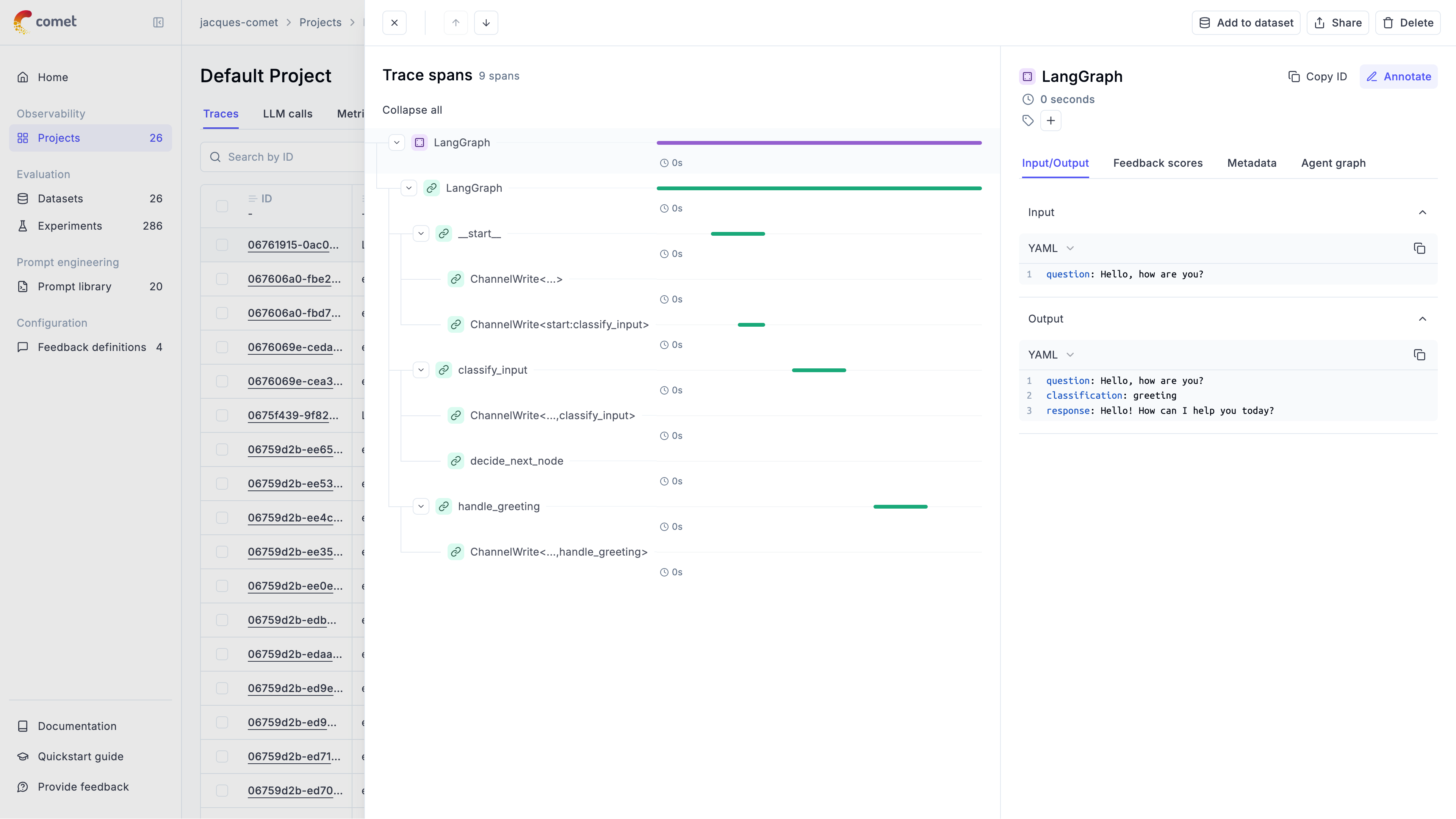
Track the agent definition
If you are using out LangGraph integration, you can also track the agent definition by passing in the graph
argument to the OpikTracer
callback:
This allows you to view the agent definition in the Opik dashboard:
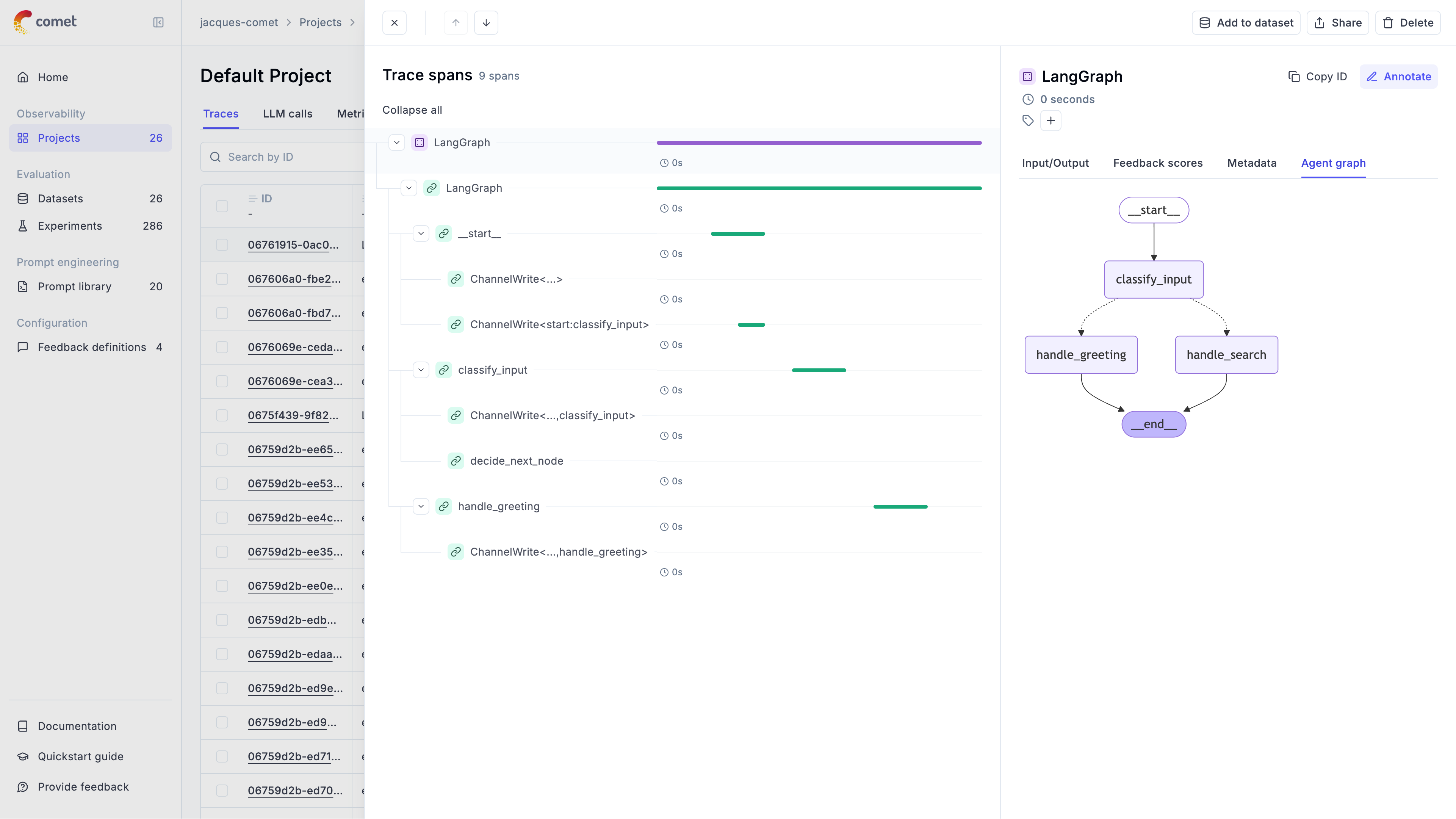