LangGraph
Opik provides a seamless integration with LangGraph, allowing you to easily log and trace your LangGraph-based applications. By using the OpikTracer
callback, you can automatically capture detailed information about your LangGraph graph executions during both development and production.
Getting Started
To use the OpikTracer
with LangGraph, you’ll need to have both the opik
and langgraph
packages installed. You can install them using pip:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
Using the OpikTracer
You can use the OpikTracer
callback with any LangGraph graph by passing it in as an argument to the stream
or invoke
functions:
Once the OpikTracer is configured, you will start to see the traces in the Opik UI:
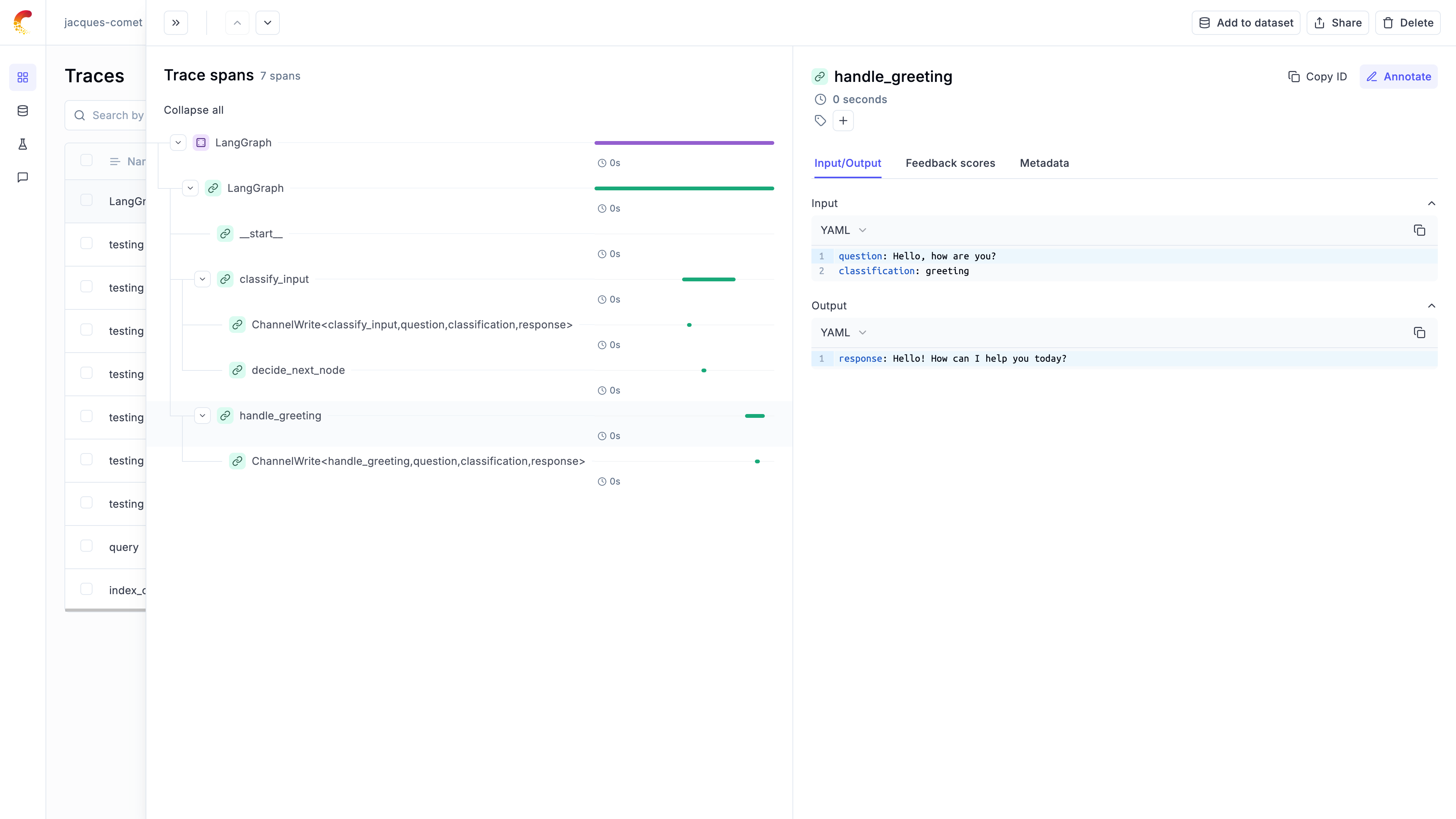
Updating logged traces
You can use the OpikTracer.created_traces
method to access the trace IDs collected by the OpikTracer callback:
These can then be used with the Opik.log_traces_feedback_scores
method to update the logged traces.
Advanced usage
The OpikTracer
object has a flush
method that can be used to make sure that all traces are logged to the Opik platform before you exit a script. This method will return once all traces have been logged or if the timeout is reach, whichever comes first.