Llama Index
LlamaIndex is a flexible data framework for building LLM applications:
LlamaIndex is a “data framework” to help you build LLM apps. It provides the following tools:
- Offers data connectors to ingest your existing data sources and data formats (APIs, PDFs, docs, SQL, etc.).
- Provides ways to structure your data (indices, graphs) so that this data can be easily used with LLMs.
- Provides an advanced retrieval/query interface over your data: Feed in any LLM input prompt, get back retrieved context and knowledge-augmented output.
- Allows easy integrations with your outer application framework (e.g. with LangChain, Flask, Docker, ChatGPT, anything else).
Getting Started
To use the Opik integration with LlamaIndex, you’ll need to have both the opik
and llama_index
packages installed. You can install them using pip:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
Using the Opik integration
To use the Opik integration with LLamaIndex, you can use the set_global_handler
function from the LlamaIndex package to set the global tracer:
Now that the integration is set up, all the LlamaIndex runs will be traced and logged to Opik.
Example
To showcase the integration, we will create a new a query engine that will use Paul Graham’s essays as the data source.
First step: Configure the Opik integration:
Second step: Download the example data:
Third step:
Configure the OpenAI API key:
Fourth step:
We can now load the data, create an index and query engine:
Given that the integration with Opik has been set up, all the traces are logged to the Opik platform:
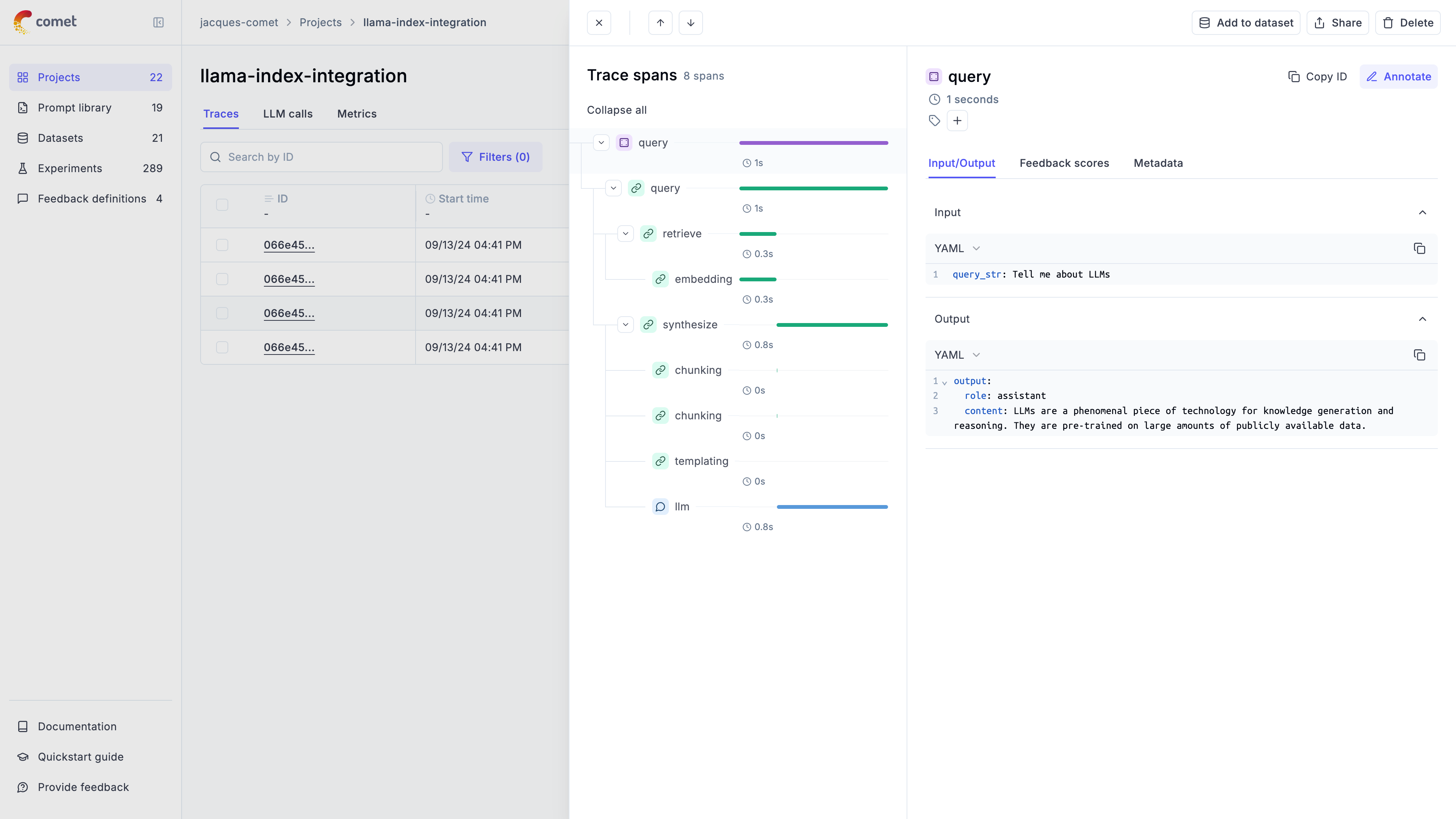