Export data
When working with Opik, it is important to be able to export traces and spans so that you can use them to fine-tune your models or run deeper analysis.
You can export the traces you have logged to the Opik platform using:
- Using the Opik SDK: You can use the
Opik.search_traces
andOpik.search_spans
methods to export traces and spans. - Using the Opik REST API: You can use the
/traces
and/spans
endpoints to export traces and spans. - Using the UI: Once you have selected the traces or spans you want to export, you can click on the
Export CSV
button in theActions
dropdown.
The recommended way to export traces is to use the
Opik.search_traces
and
Opik.search_spans
methods
in the Opik SDK.
Using the Opik SDK
Exporting traces
The Opik.search_traces
method allows you to both export all the traces in a project or search for specific traces and export them.
Exporting all traces
To export all traces, you will need to specify a max_results
value that is higher than the total number of traces in your project:
Search for specific traces
You can use the filter_string
parameter to search for specific traces:
The filter_string
parameter should be a string in the following format:
where:
<COLUMN>
: The column name to filter on, these can be:name
input
output
start_time
end_time
metadata
feedback_scores
tags
usage.total_tokens
usage.prompt_tokens
usage.completion_tokens
.
<OPERATOR>
: The operator to use for the filter, this can be=
,!=
,>
,>=
,<
,<=
,contains
,not_contains
. Not that not all operators are supported for all columns.<VALUE>
: The value to use in the comparison to<COLUMN>
. If the value is a string, you will need to wrap it in double quotes.
You can add as many and
clauses as required.
If a <COLUMN>
item refers to a nested object, then you can use the
dot notation to access contained values by using its key. For example,
you could use:
"feedback_scores.accuracy > 0.5"
Here are some full examples of using filter_string
values in searches:
If your feedback_scores
key contains spaces, you will need to wrap
it in double quotes:
'feedback_score."My Score" > 0'
If the feedback_score
key contains both spaces and double quotes, you will need to escape the double quotes as ""
:
'feedback_score."Score ""with"" Quotes" > 0'
or by using different quotes, surrounding in triple-quotes, like this:
'''feedback_scores.'Accuracy "Happy Index"' < 0.8'''
Exporting spans
You can export spans using the Opik.search_spans
method. This methods allows you to search for spans based on trace_id
or based on a filter string.
Exporting spans based on trace_id
To export all the spans associated with a specific trace, you can use the trace_id
parameter:
Search for specific spans
You can use the filter_string
parameter to search for specific spans:
The filter_string
parameter should follow the same format as the filter_string
parameter in the
Opik.search_traces
method as defined above.
Using the Opik REST API
To export traces using the Opik REST API, you can use the /traces
endpoint and the /spans
endpoint. These endpoints are paginated so you will need to make multiple requests to retrieve all the traces or spans you want.
To search for specific traces or spans, you can use the filter
parameter. While this is a string parameter, it does not follow the same format as the filter_string
parameter in the Opik SDK. Instead it is a list of json objects with the following format:
The filter
parameter was designed to be used with the Opik UI and has therefore limited flexibility. If you need
more flexibility, please raise an issue on GitHub so we can help.
Using the UI
To export traces as a CSV file from the UI, you can simply select the traces or spans you wish to export and click on Export CSV
in the Actions
dropdown:
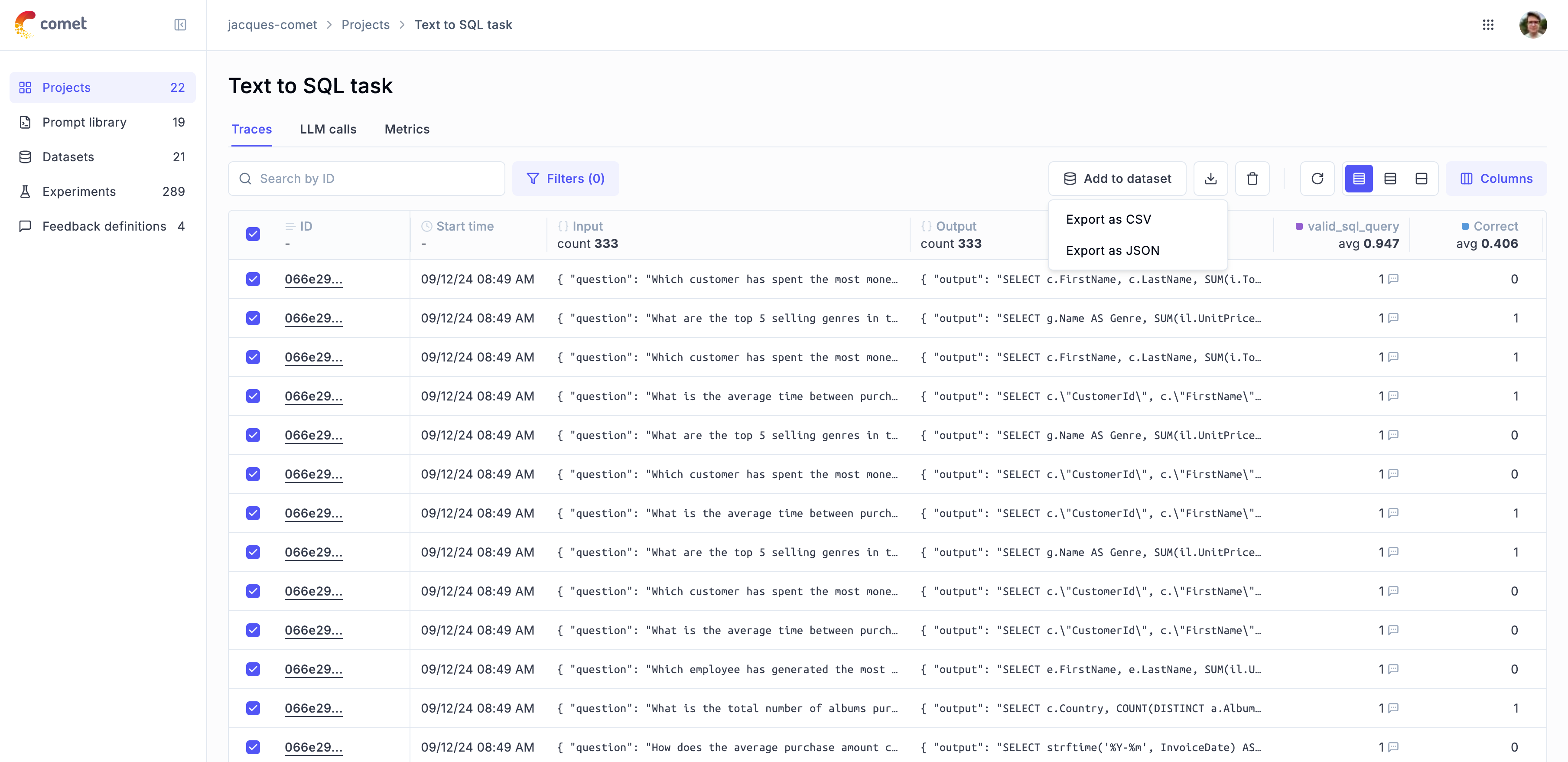
The UI only allows you to export up to 100 traces or spans at a time as it is linked to the page size of the traces table. If you need to export more traces or spans, we recommend using the Opik SDK.