Groq
Groq is Fast AI Inference.
Getting Started
Configuring Opik
To start tracking your Groq LLM calls, you can use our LiteLLM integration. You’ll need to have both the opik
and litellm
packages installed. You can install them using pip:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
If you’re unable to use our LiteLLM integration with Groq, please open an issue
Configuring Groq
In order to configure Groq, you will need to have:
- Your Groq API Key: You can create and manage your Groq API Keys on this page.
Once you have these, you can set them as environment variables:
Logging LLM calls
In order to log the LLM calls to Opik, you will need to create the OpikLogger callback. Once the OpikLogger callback is created and added to LiteLLM, you can make calls to LiteLLM as you normally would:
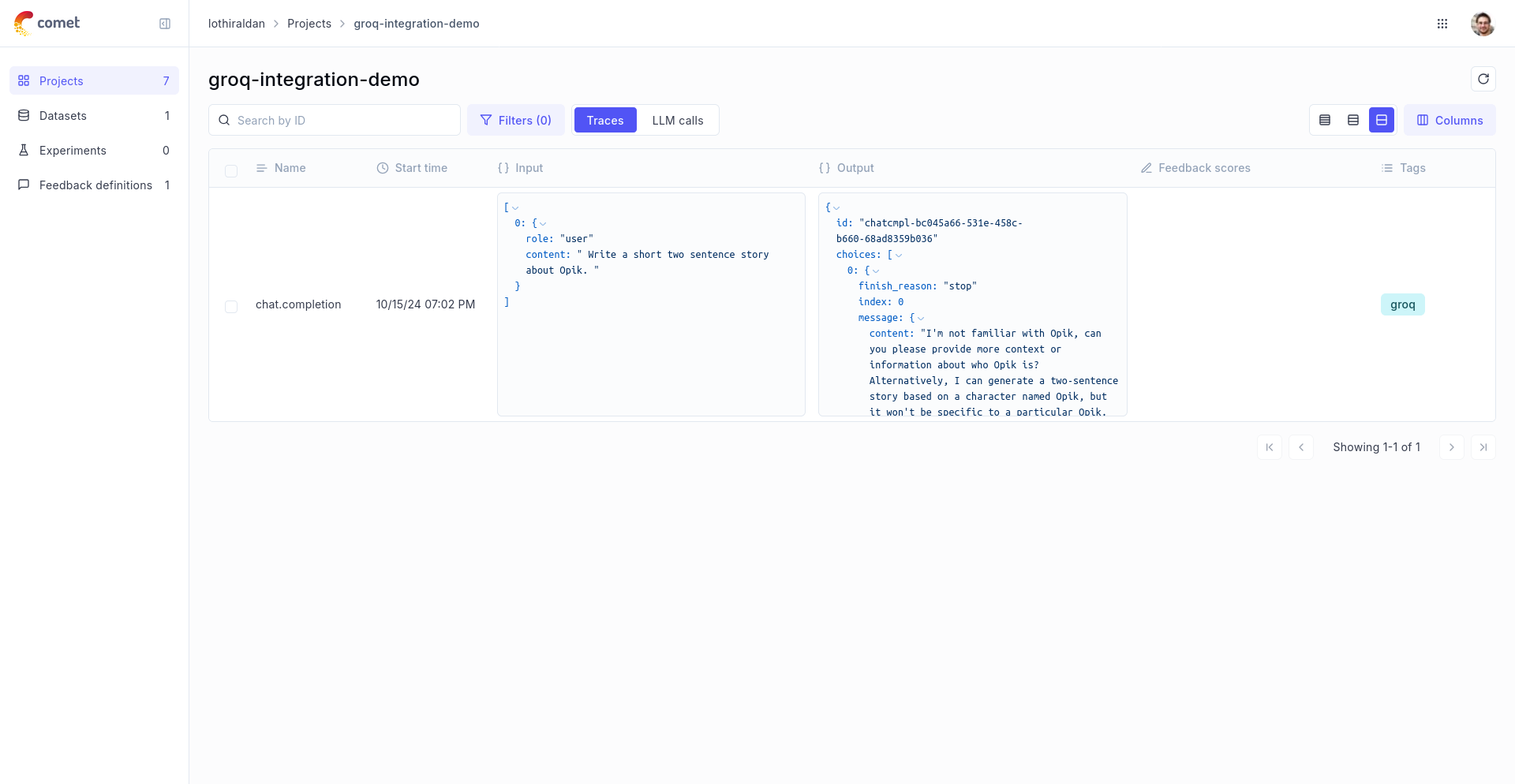
Logging LLM calls within a tracked function
If you are using LiteLLM within a function tracked with the @track
decorator, you will need to pass the current_span_data
as metadata to the litellm.completion
call:
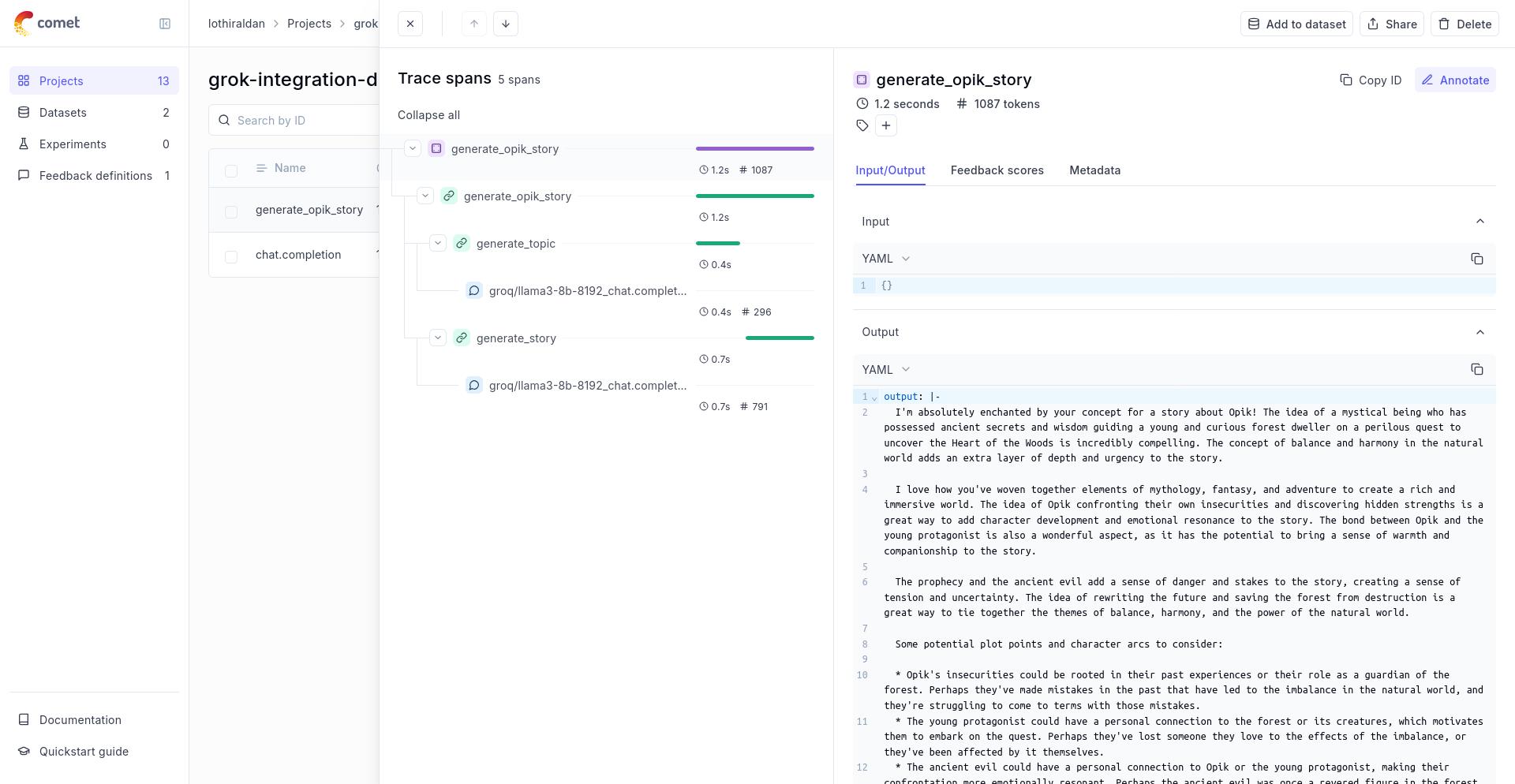