Crewai
CrewAI is a cutting-edge framework for orchestrating autonomous AI agents.
Opik integrates with CrewAI to log traces for all CrewAI activity.
Getting started
First, ensure you have both opik
and crewai
installed:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
Logging CrewAI calls
To log a CrewAI pipeline run, you can use the track_crewai
. This callback will log each CrewAI call to Opik:
Each run will now be logged to the Opik platform:
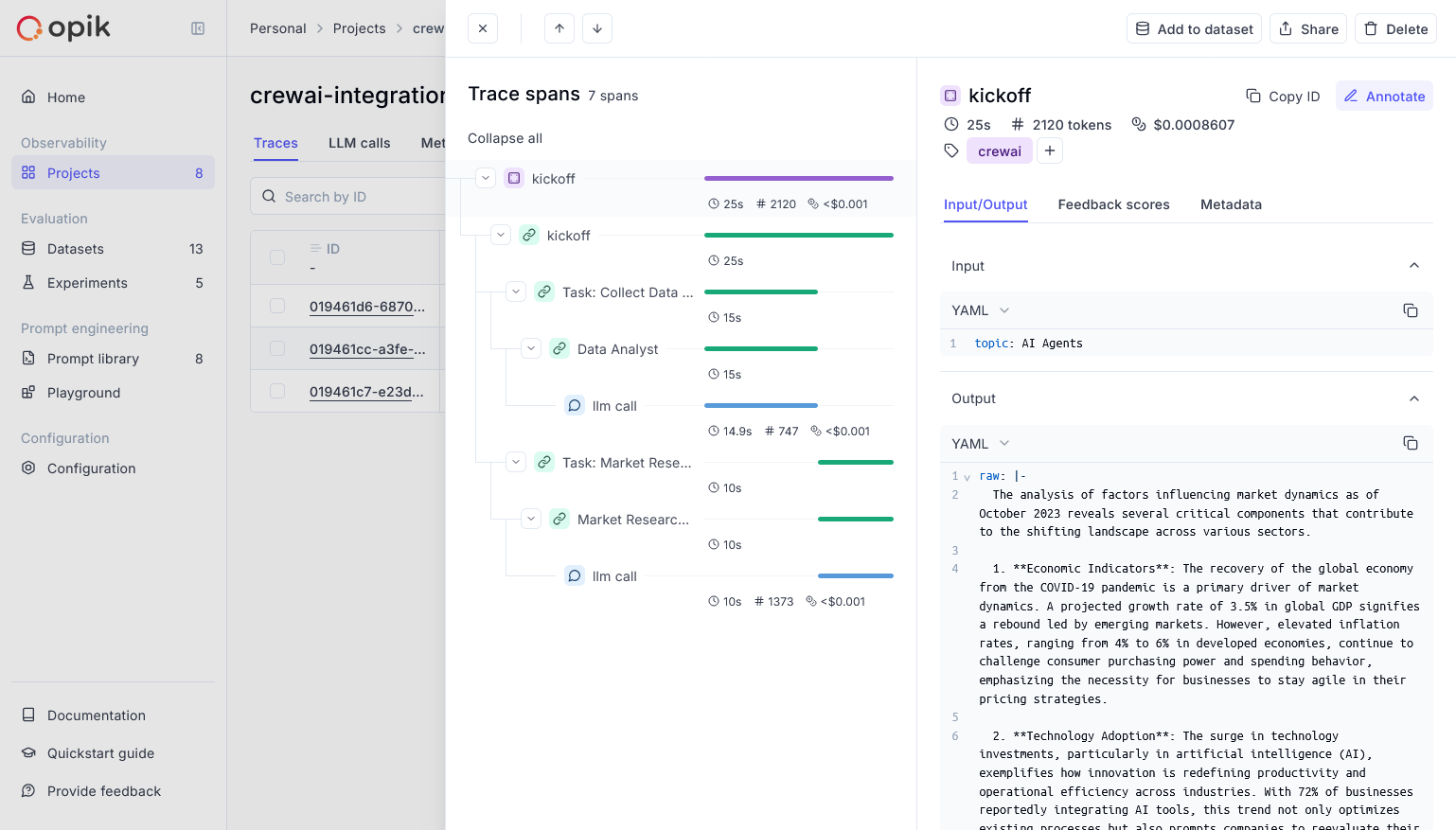