Bedrock
AWS Bedrock is a fully managed service that provides access to high-performing foundation models (FMs) from leading AI companies like AI21 Labs, Anthropic, Cohere, Meta, Mistral AI, Stability AI, and Amazon through a single API.
This guide explains how to integrate Opik with the Bedrock Python SDK. By using the track_bedrock
method provided by opik, you can easily track and evaluate your Bedrock API calls within your Opik projects as Opik will automatically log the input prompt, model used, token usage, and response generated.
Getting Started
Configuring Opik
To start tracking your Bedrock LLM calls, you’ll need to have both the opik
and boto3
. You can install them using pip:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
Configuring Bedrock
In order to configure Bedrock, you will need to have:
- Your AWS Credentials configured for boto, see the following documentation page for how to set them up.
- Access to the model you are planning to use, see the following documentation page how to do so.
Once you have these, you can set create your boto3 client:
Logging LLM calls
In order to log the LLM calls to Opik, you will need to create the wrap the boto3 client with track_bedrock
. When making calls with that wrapped client, all calls will be logged to Opik:
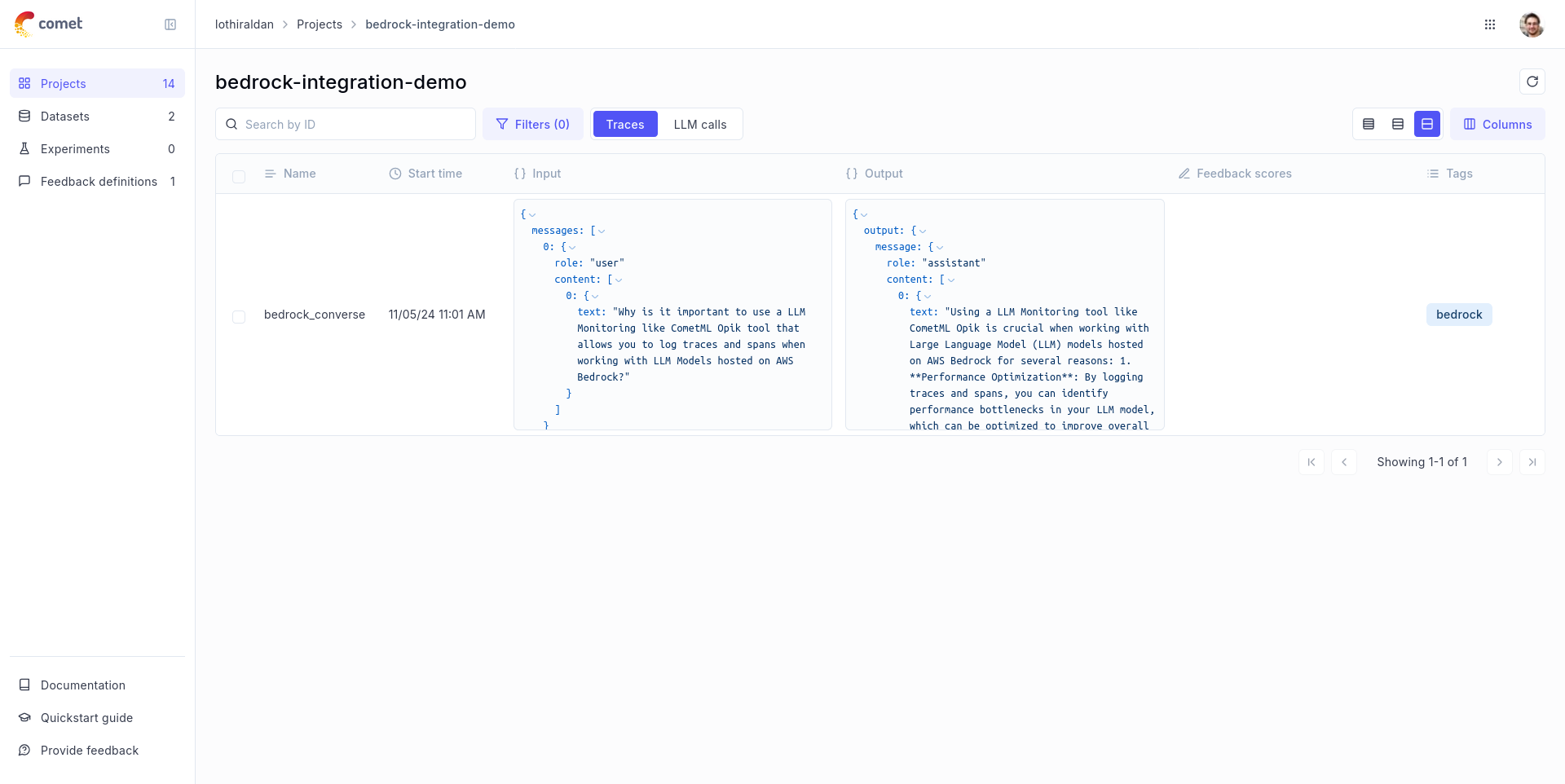