Instructor
Instructor is a Python library for working with structured outputs for LLMs built on top of Pydantic. It provides a simple way to manage schema validations, retries and streaming responses.
Getting started
First, ensure you have both opik
and instructor
installed:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or
if you are using the Cloud platform your API key:
Using Opik with Instructor library
To use Opik with Instructor, we are going to rely on the existing Opik integrations with popular LLM providers.
For all the integrations, we will first add tracking to the LLM client and then pass it to the Instructor library:
Thanks to the track_openai
method, all the calls made to OpenAI will be logged to the Opik platform. This approach also works well if you are also using the opik.track
decorator as it will automatically log the LLM call made with Instructor to the relevant trace.
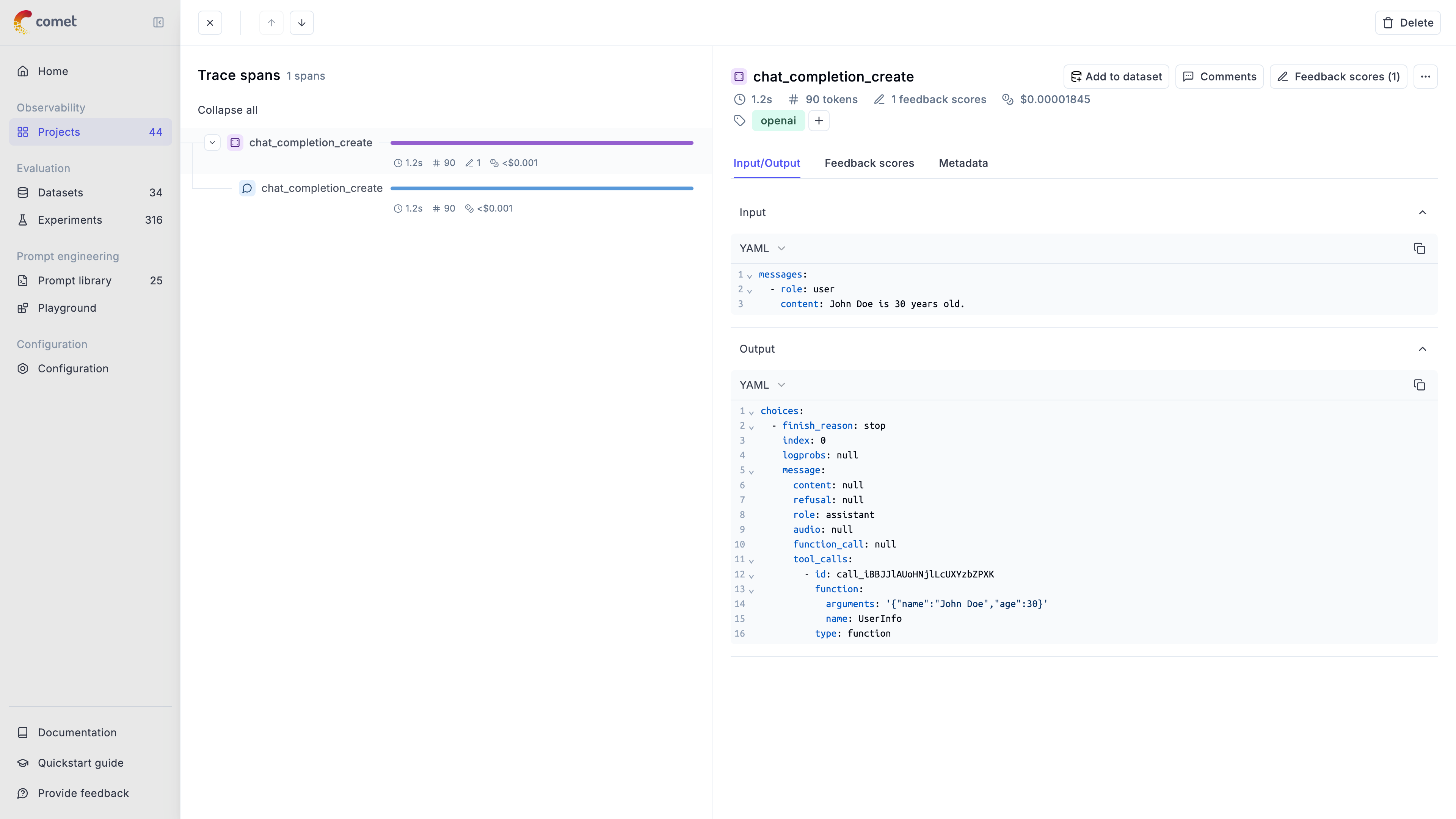
Supported LLM providers
Opik supports the OpenAI, Anthropic and Gemini LLM providers, feel free to create a Github issue if you would like us to support more providers.
OpenAI
Anthropic
Gemini
You can read more about how to use the Instructor library in their documentation.