Log traces
If you are just getting started with Opik, we recommend first checking out the Quickstart guide that will walk you through the process of logging your first LLM call.
LLM applications are complex systems that do more than just call an LLM API, they will often involve retrieval, pre-processing and post-processing steps. Tracing is a tool that helps you understand the flow of your application and identify specific points in your application that may be causing issues.
Opik’s tracing functionality allows you to track not just all the LLM calls made by your application but also any of the other steps involved.
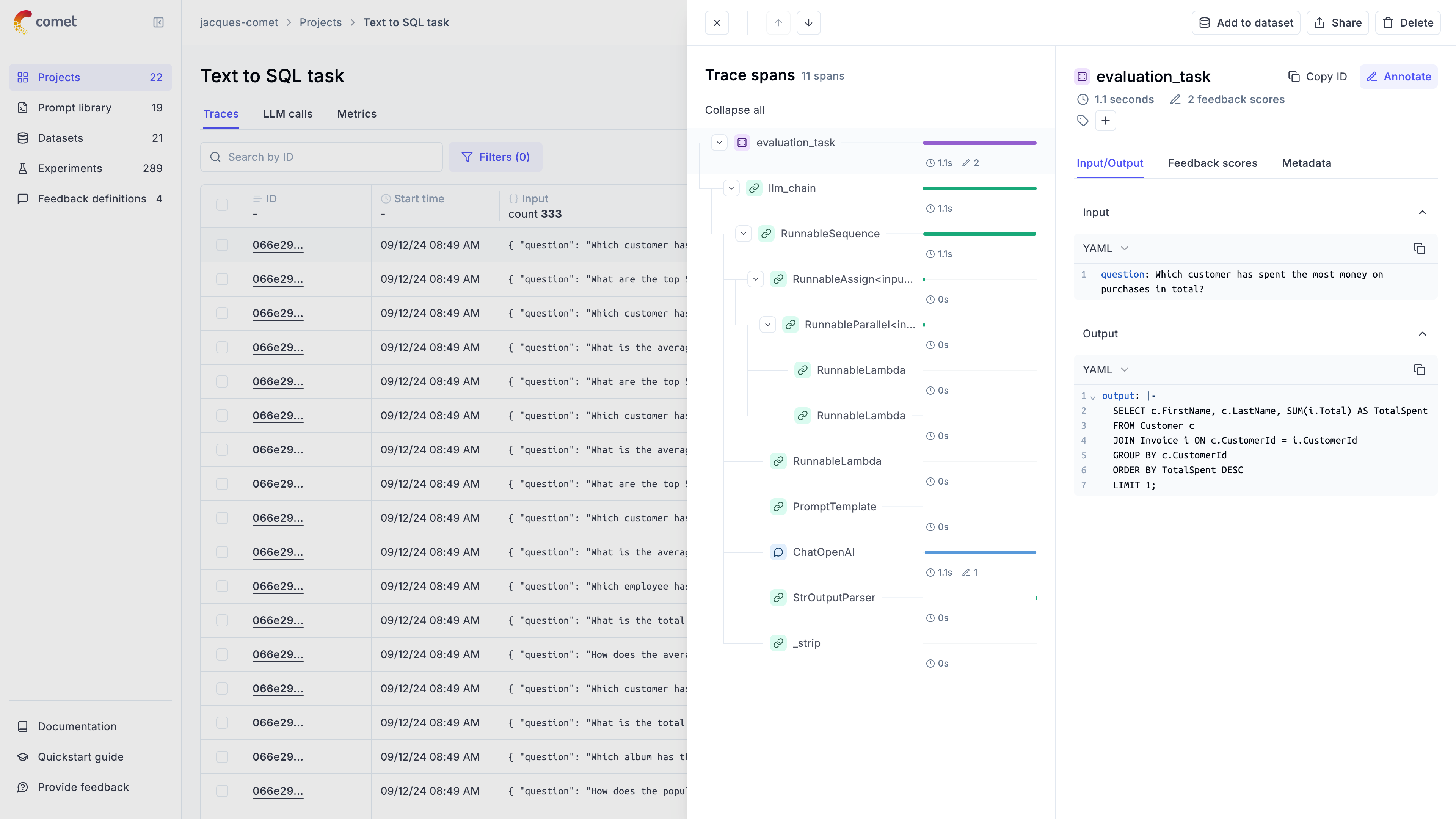
Opik provides different ways to log your LLM calls and traces to the platform:
- Using one of our integrations: This is the easiest way to get started.
- Using the
@track
decorator: This allows you to track not just LLM calls but any function call in your application, it is often used in conjunction with the integrations. - Using the Python SDK: This allows for the most flexibility and customizability and is recommended if you want to have full control over the logging process.
- Using the Opik REST API: If you are not using Python, you can use the REST API to log traces to the platform. The REST API is currently in beta and subject to change.
Logging with the Python SDK
In order to use the Opik Python SDK, you will need to install it and configure it:
Command Line
Jupyter Notebook
Opik is open-source and can be hosted locally using Docker, please refer to the self-hosting guide to get started. Alternatively, you can use our hosted platform by creating an account on Comet.
Using an integration
When using one of Opik’s integration you will simply need to add a couple of lines of code to your existing application to track your LLM calls and traces. There are integrations available for many of the most popular LLM frameworks and libraries.
Here is a short overview of our most popular integrations:
OpenAI
LangChain
LlamaIndex
First let’s install the required dependencies:
By wrapping the OpenAI client in the track_openai
function, all calls to the OpenAI API will be logged to the Opik platform:
If you are using a framework that Opik does not integrate with, you can raise a feature request on our Github repository.
If you are using a framework that Opik does not integrate with, we recommed you use the opik.track
function decorator.
Using function decorators
Using the opik.track
decorator is a great way to add Opik logging to your existing LLM application. We recommend using this
method in conjunction with one of our integrations for the most seamless experience.
When you add the @track
decorator to a function, Opik will create a span for that function call and log the input parameters and function output
for that function. If we detect that a decorated function is being called within another decorated function, we will create a nested span for the
inner function.
Decorating your code
You can add the @track
decorator to any function in your application and track not just LLM calls but also any other steps in your application:
The @track
decorator will only track the input and output of the decorated function. If you are using OpenAI, we recommend you also use the track_openai
function to track the LLM
call as well as token usage:
Scoring traces
You can log feedback scores for traces using the opik_context.update_current_trace
function. This can be useful if
there are some metrics that are already reported as part of your chain or agent:
You don’t have to manually log feedback scores, you can also define LLM as a Judge metrics in Opik that will score traces automatically for you.
You can learn more about this feature in the Online evaluation guide.
Logging additional data
As mentioned above, the @track
decorator only logs the input and output of the decorated function. If you want to log additional data, you can use the
update_current_span
function and update_current_trace
function to manually update the span and trace:
You can learn more about the opik_context
module in the opik_context reference docs.
Configuring the project name
You can configure the project you want the trace to be logged to using the project_name
parameter of the @track
decorator:
If you want to configure this globally for all traces, you can also use the environment variable:
This will block the processing until the data is finished being logged.
Flushing the trace
You can ensure all data is logged by setting the flush
parameter of the @track
decorator to True
:
Disabling automatic logging of function input and output
You can use the capture_input
and capture_output
parameters of the @track
decorator to disable the automatic logging of the function input and output:
You can then use the opik_context
module to manually log the trace and span attributes.
Disable all tracing
You can disable the logging of traces and spans using the enviornment variable OPIK_TRACK_DISABLE
, this will turn off the logging for all function decorators:
Using the low-level Opik client
If you want full control over the data logged to Opik, you can use the Opik
client to log traces, spans, feedback scores and more.
Logging traces and spans
Logging traces and spans can be achieved by first creating a trace using Opik.trace
and then adding spans to the trace using the Trace.span
method:
It is recommended to call trace.end()
and span.end()
when you are finished with the trace and span to ensure that
the end time is logged correctly.
Logging feedback scores
You can log scores to traces and spans using the log_traces_feedback_scores
and log_spans_feedback_scores
methods:
If you want to log scores to traces or spans from within a decorated function, you can use the update_current_trace
and update_current_span
methods instead.
Ensuring all traces are logged
Opik’s logging functionality is designed with production environments in mind. To optimize performance, all logging operations are executed in a background thread.
If you want to ensure all traces are logged to Opik before exiting your program, you can use the opik.Opik.flush
method:
Copying traces to a new project
You can copy traces between projects using the copy_traces
method. This
method allows you to move traces from one project to another without having to re-log them.
By default, the copy_traces
method will not delete the traces in the source project. You can optionally set
the delete_original_project
parameter to true
to delete the traces in the source project after copying them.
This is not recommended, instead we recommend moving the traces and once everything has been migrated you can delete the source project from the UI.
Logging with the JS / TS SDK
You can log your LLM calls using the Opik typescript SDK opik
. We are actively adding functionality to the TypeScript SDK,
if you have any suggestions on how we can improve it feel free to open an issue on GitHub.
You can find the reference documentation for the opik
typescript SDK here.
Using the low-level Opik client
The easiest way to log your LLM calls is using the low-level Opik client. We do have support for decorators but this is currently considered experimental.
Setting up the Opik client
The first step is to install the Opik library:
Once the library is installed, you can initialize the Opik client with explicit configuration:
Or using environment variables instead:
If you are using the self-hosted Opik platform, you can replace the host with
http://localhost:5173/api
and remove the workspaceName
parameter.
Logging traces and spans
Once the Opik client is set up, you can log your LLM calls by adding spans to the trace:
Decorators
On calling process()
it’ll create a trace and create all the spans for each tracked function called in this function
Class method decorators (TypeScript)
TypeScript started supporting decorators from version 5 but it’s use is still not widespread. The Opik typescript SDK also supports decorators but it’s currently considered experimental.
Using the REST API
The Opik REST API is currently in beta and subject to change, if you encounter any issues please report them to the Github.
The documentation for the Opik REST API is available here.